Entity Framework (.NET 4.0)
Entity Framework is a feature of ADO.NET which allows developers to code
applications which access data using a data source intedependent way. Entity
Framework provides the same conceptual model irrespective of the type of
underlying data. Note: this is different to LINQ to SQL classes, as LINQ to SQL
provides object mapping only to SQL database systems.
Using Entity Framework the conceptual object model for your data can be accessed
using LINQ in just the same way as LINQ to SQL.
Creating the inital model
We will use the same scenario as the LINQ to SQL
example. To recap - the database is based on a bookshop with the two tables
below:
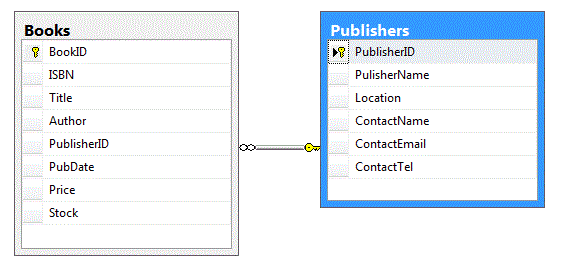
To create our O/RM model we add a New Item of type ADO.NET Entity Data Model.
The wizard dialog allows us to choose our existing database connection and
generate the model automatically. The resulting classes look like this:
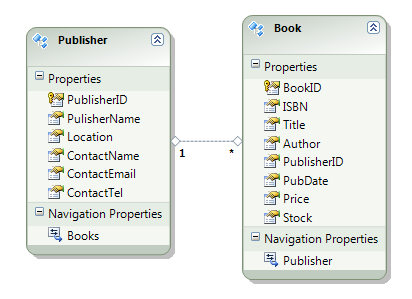
Note: The wizard has generated classes based on the two tables. Not only that it
creates a DataContext class with properties for each table in our database. The
data content class is called BookshopDataContext and when we instantiate it, we
can use it's methods and properties to interact directly with the database. Note
also: the each class has a property for related table from the existing
sdatabase relationships - the Publisher class has a collection property called
books which contains a list of all books for the particular publisher when a
Publisher object is instantiated.
Querying our database
If we want to retrieve data from the database we first create an instance of our
data contenct class and then we can use LINQ syntax to perform the query against
objects in our database. For example, to return a list of Books by Luke
Rhinehart the code would be:
1 |
BookshopDataContext db =
new
BookshopDataContext();
|
3 |
var lukesbooks = from book
in db.Books
|
4 |
where
book.Author == "Luke Rhinehart" |
Of course we could use the LINQ extension methods, instead of LINQ query syntax:
1 |
BookshopDataContext db =
new
BookshopDataContext();
|
3 |
var lukesbooks = db.Products.Where( book =>
book.Author == "Luke Rhinehart" ); |
Modifying data
If we retrieve data from the database we can modify its values directly. Once we
want to update the database we call the SubmitChanges method wich commits all
previous transactions to the database. Each modelled table (e.g. Books) has an
Add method which allows it to insert a new record. Again, the insert is only
committed once we call the SubmitChanges method. For example, to add a new
publisher we would:
01 |
BookshopDataContext db =
new
BookshopDataContext();
|
03 |
Publisher pub =
new Publisher {
|
04 |
PublisherName =
"Wiley" ,
|
06 |
ContactName =
"Joe Bloggs" ,
|
08 |
ContactTel =
"02345 789543" };
|
10 |
db.Publishers.InsertOnSubmit(pub);
|
To retrieve the record for Wiley and update the telephone number we would:
1 |
BookshopDataContext db =
new
BookshopDataContext();
|
3 |
var pub = db.Publishers.Single(p => p.PublisherName
== "Wiley" );
|
5 |
pub.ContactTel =
"08705 123456" ;
|
To delete the record for Wiley from the database we would:
1 |
BookshopDataContext db =
new
BookshopDataContext();
|
3 |
var pub = db.Publishers.Single(p => p.PublisherName
== "Wiley" );
|
5 |
pub.Publishers.Remove(pub);
|
Further actions
If our database contains stored procedures we can include these in our Entity
Framework model and the methods will be available directly to the developer. We
can also take advantage of other server side processing, such as paging of query
results.
The deferred execution model means that database handling is done efficiently.
The extension methods Skip and Take use this feature and allow the developer to
deliver paged results with minimal overhead in the local application, as the
pagination is done on the database server.