Sending an Email
Let's try an create an application which performs a useful function.
Most web applications rely on using email to perform certain functions. For example,
an eCommerce web site will send a confirmation email when an order has been processed,
and probably when the order has been dispatched. When a user subscribes to a web
site they may request their password be sent by email if they forget it. Fortunately,
ASP.NET 2.0 has and inbuilt set of Web controls for handling the creation and sending
of email. They also need to be handled using script code, so they provide a useful
exercise in writing C# scripts.
We will develop three simple email applications:
- Sending a standard message automatically (FixedMail)
- Sending a simple user configured message to a fixed email account (FeedbackMail)
- Creating a generic email sender (SendMail)
If we look at the C# code produced by default when you create a new Web Form you
will see that each page makes use of a set of external resources. The code below
is generated automatically when you create a new Web Form:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
}
The 'using' clauses at the top of the code tell .NET to make available additional
libraries. In the case of a simple web form these will include libraries for working
with HTML, Web Controls and basic system configuration and security features. When
we want to perform more specialised functions within our pages we need to include
the names of the appropriate libraries. E.g. for working with images we may use:
'System.Drawing'. .NET has a large set of built in libraries which you can build
extra functionality into your web forms, the most important of which are probably
the database connectivity libraries.
For the email enabled pages in this session to
work we must make sure that there is a using clause at the top of the each *.aspx.cs file,
as follows:
using System.Net.Mail;
This process is simplified a little by the way Visual Studio 2008 helps
the developer. If you type the name of something which isn't available
in the current libraries you can right click it and choose 'Resolve' and
it will pop up the library entry and include it in your file when you
select it.
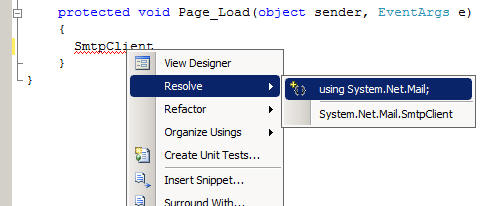
FixedMail
Many web applications automatically generate an email to the user to provide information
of many sorts. For example, to send the user their password, to confirm receipt
of an order, or to send an email newsletter (to a subscriber). The first application
is a simplified example of sending a fixed email to a user. The user will usually
be specified from a database, but in this case the email address is simply typed
into a textbox on a simple web form.
The SmtpClient class has a Send method which in one form takes as parameters
four strings. The first string is the senders email address, the second is the recipients
email address, the third is the subject of the email and the fourth is the body
of the email message. A simple web form can be built with a text box for the email
address of the recipient, and a button to cause an email to be sent to the person.
Assume there is a button called butSend and a TextBox called txtEMailAddress. The
code to send a fixed message to the person identified in the TextBox would be:
protected void butSend_Click(object sender, EventArgs e)
{
SmtpClient Mail = new SmtpClient("smtp.myISP.com");
Mail.Send("[email protected]", txtEMailAddress.Text,
"Test message", "Hello from admin");
}
Note: the SmtpClient constructor needs the name of a valid SMTP server for it to
work properly. You can use the same SMTP server that you have configured for your
Internet Service Provider, or the one configured for your email account in Outlook
or Outlook Express. As a last resort, you can also install an SMTP server on your
own machine (try www.pmail.com for the Mercury email server) and use your own IP
address.
The above example is shown in application
FixedMail
and makes use of the SMTP server for the web server hosting this tutorial. Download
a
ZIP version of FixedMail.
Download, unzip, open and run FixedMail, type in your own email address and click the Send Mail Message
button.
Now check your own email to see if the message has arrived. Note: there may be a short delay, so if you do not receive the message first time check your account
later. Don't forget to modify the SMTP server's address to match your
environment.
Note: if your ISP requires a secure login for the SMTP Server you will
need to modify the above code as follows. Don't forget to change your
SMTP server and username and password to match your ISP:
protected void butSend_Click(object sender, EventArgs e)
{
SmtpClient Mail = new SmtpClient("smtp.myISP.com");
mail.Credentials =
new NetworkCredential("username", "password");
Mail.Send("[email protected]", txtEMailAddress.Text,
"Test message", "Hello from admin");
}
IMPORTANT: You should never send an email impersonating another
user; this is a practice used by the worst sort of Internet fraudsters. A secure
mail server will refuse to send an email which doesn't come from the same domain,
but some systems are not secure. It is usual for a web application to send automatic
email from an internal generic account ([email protected] in the example above) which
is configured not to receive email. This account will usually be configured by the
webmaster in liaison with the developer.
FeedbackMail
A lot of sites allow clients to send feedback in the form of an email. There has
been a recent trend towards using a database to collect the information directly,
however. In this scenario we are providing a user feedback facility which will fire
off an email from the server to a site coordinator who will be responsible for monitoring
and acting on user feedback.
Let's use the simple version of the SmtpMail.Send method to allow a user to fire
off some feedback to the site coordinator.
Build a web form with three textboxes. One will be for the senders email address
(txtSender). The second will be the subject of the email (txtSubject), and the third
will be the body of the message (txtBody). The recipient will be the site administrator
(in this case [email protected]). Add a button
called butSend. In the event handler for clicking the button type the following
code:
protected void butSend_Click(object sender, EventArgs e)
{
SmtpClient Mail = new SmtpClient("smtp.myISP.com");
Mail.Send("[email protected]", txtSender.Text,
txtSubject.Text,txtBody.Text);
}
Don't forget to put the using clause at the top of the .aspx.cs file to link to
the System.Net.Mail library. If you want to try out application FeedbackMail you
will need to replace the email address '[email protected]' with an email address
of your own, so that you can check that the system works. For testing purposes you
can put a fictitious email address for the sender, as you will be the recipient.
You will need to check your email system for an email from the fictitious account.
Download a
ZIP version of FeedbackMail.
SendMail
The previous examples used the basic variant of the SmtpMail.Send method. The other
method takes a single parameter of type MailMessage.
MailMessage
is a class with properties which allow you to specify all the fields for an email
message, not just From, To, Subject and Body. With a MailMessage object you can
easily specify fields such as Cc and Bcc fields as well as attachments.
It also handle multiple recipients.
Now we build a form with text boxes for From, To, CC, Bcc, Subject and Body, called
txtTo, txtFrom, txtCC, txtBCC, txtSubject and txtBody. Note: the 'To' field can
contain a list of email address separated by commas, and the 'CC' and 'Bcc' fields
may be empty, but could contain lists of email addresses. The code will need to
check if there are any values for CC or Bcc before trying to add them to the MailMessage
object.
The send button has the following code:
protected void butSend_Click(object sender, EventArgs e)
{
MailMessage Mess = new MailMessage();
Mess.From = new MailAddress(txtFrom.Text);
Mess.To.Add(txtTo.Text);
if (txtCC.Text != "")
{
Mess.CC.Add(txtCC.Text);
}
if (txtBCC.Text != "")
{
Mess.Bcc.Add(txtBCC.Text);
}
Mess.Subject = txtSubject.Text;
Mess.Body = txtBody.Text;
SmtpClient mail = new SmtpClient("smtp.mydomain.com");
mail.Send(Mess);
}
Download a
ZIP version of SendMail.