Customising pages
A basic web page design will often follow some aesthetic pleasing set of styles. The whole site will use a set of style elements encompassing all of the elements on the web page, from text, to headings, to buttons etc. A simple Cascading Style sheet can handle this requirement quite easily, and is a well understood technique in 'traditional' web page design.
The look and feel of a user interface is often a cause for concern. Take for example the Windows user interface; many users will modify their desktop to use one of the custom themes, or even customise the colours etc. themselves. Sometimes this is done for personal aesthetic reasons, but in other cases this may be prompted by usability criteria, for example, switching to special colour combinations for dyslexic users. Traditional web design techniques have made it possible for users to change appearance by linking alternative style sheets using client side scripts. ASP.NET 2.0 introduces an alternative technique for server side style changes using what Microsoft refer to as Themes and Skins.
Note: Skins are not an alternative to stylesheets as they only apply to .NET web
controls. If you need to control the appearance of plain XHTML elements as well
you should do this using a CSS style sheet.
Skins
A skin is a collection of style values for an individual ASP.NET web control. For example, an ASP Label could have a font defined as well as font size, style and colour. This collection of style changes can be save in a .skin file so that it can be used when you design your web pages. A .skin file can contain lots of skins for a set of controls. For example, an ASP Label may be used on a web page for headings, ordinary text and emphasis. The formatting for each of these will be different. A default skin would be used for ordinary text, but the heading and emphasis skins would each be given a SkinID, such as 'Heading' and 'Emphasis'. Although skin files are nothing like CSS files, the .NET web server delivers the web pages as standard XHTML with standard CSS syntax.
A skin looks very much like the ASP code for the particular control. The difference is that there is no ID attribute or actual data values. A skin can be created by producing a sample web page with formatted controls on it. The controls can be pasted into the skin file and edited to remove the ID and actual values. A SkinID attribute can be added if you need different skins for one control.
For example, the following code is cut from a page with three differently formatted labels:
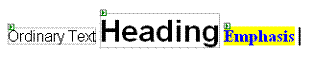
coded as follows:
<asp:Label ID="Label1" Runat="server"
Text="Ordinary Text"
Font-Names="Verdana,Helvetica,Sans-Serif">
</asp:Label>
<asp:Label ID="Label2" Runat="server"
Text="Heading"
Font-Names="Verdana,Helvetica,Sans-Serif"
Font-Bold="True" Font-Size="X-Large">
</asp:Label>
<asp:Label ID="Label3" Runat="server"
Text="Emphasis" Font-Bold="True"
Font-Size="Larger" ForeColor="Blue"
BackColor="Yellow">
</asp:Label>
copy this into the .skin file and remove the ID and Text attributes. Add a SkinID attribute for the heading and emphasis styles, resulting in this .skin file
<asp:Label Runat="server"
Font-Names="Verdana,Helvetica,Sans-Serif">
</asp:Label>
<asp:Label SkinID="Heading" Runat="server"
Font-Names="Verdana,Helvetica,Sans-Serif"
Font-Bold="True" Font-Size="X-Large">
</asp:Label>
<asp:Label SkinID="Emphasis" Runat="server"
Font-Bold="True" Font-Size="Larger"
ForeColor="Blue" BackColor="Yellow">
</asp:Label>
Now, if you add labels into a web page you can set the SkinID property for those labels which need to be headings or emphasised.
Themes
A theme is a set of skins for a web application. A web application can have more than one theme available and the developer can choose the theme before deploying the application. It is also possible for a user to switch themes and save it to their own profile so that they get the same appearance each time they use the site.
Before you can use themes in your web application you need to:
- create an App_Themes folder in your application folder
- create a named theme folder within the App_Themes folder (e.g. MyTheme)
- place your skin files in this folder.
While designing your pages you will:
- enable themes
- set the Theme name for each page to the chosen theme
- set the SkinID for each control to its appropriate value (unless you are using
the default appearance, in which case you leave the SkinID empty).
Step by Step
- Create a new C# ASP.NET Web Site called Themes.
- In the solution explorer right click the application and choose Add ASP.NET
Folder | Theme.
- Rename the Theme1 folder to MyTheme.
- Right click the MyTheme folder and select Add New Item...
- Choose Skin File and name the file Label.skin.
- Delete all the comment text form the file and then copy the text from the above example into the file and save it.
You now have a skin file with a default label format, and two named formats
(Heading and Emphasis). You can now modify the web page to use your theme and
use the different skins within it.
- Add three labels to your web page on separate lines.
- Change the text of the first one to 'This is a Heading'.
- Change the text of the second one to 'This is ordinary text'.
- Change the text of the last one to 'This should stand out'.
- Switch to source view, click the page tag at the top of the file and set the
EnableTheming property to True.
- Set the Theme property to MyTheme.
- Switch back to Design view, select the heading label and set its SkinID
property to Heading.
- Select the emphasis label and set its SkinID property to Emphasis.
- Save the project and run the application.
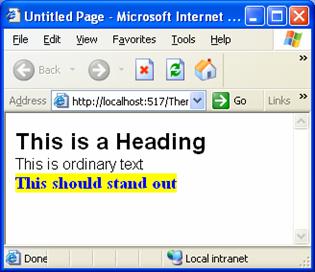
Download a
ZIP version of the Themes application.
Cascading Style Sheets
Skin files can only contain style information for Web Controls. However, Ordinary XHTML
tags can be styled using a Cascading Style Sheet stored in the Theme folder. If you
use ordinary XHTML tags you can use class names to distinguish been style requirements
on your page.
The application ThemeStyle demonstrates this. Download a
ZIP version of ThemeStyle.
The page has no web controls;
just a heading and a paragraph. The page uses a theme
called 'Standard' which only has a
CSS file in it called StyleSheet.css. The contents of the CSS file is shown below:
body
{
font-family:Arial;
}
h1
{
color:Red;
}
The result of this is that the default font for the entire page is changed to Arial, and <h1>
elements are displayed in red.
Changing themes under user control
It is straightforward to allow a user to change themes on a page. You
must first create a set of named themes for the application, and test that
they have the desired effect. If you place a listbox on a web form with a
list of the themes in it we can use the Preload event of the Page to get the
chosen theme from the listbox, and set the Theme property of the page to the
required value.
Application AdvancedThemes demonstrates this. Download a
ZIP version of AdvancedThemes.
The page contains a listbox
called ChangeTheme containing two items 'Standard' and 'Crazy'. The code for
this list box is shown below:
<asp:DropDownList ID="ChangeTheme" Runat="server"
AutoPostBack="True" >
<asp:ListItem Text="Standard" Value="Standard">
</asp:ListItem>
<asp:ListItem Text="Crazy" Value="Crazy">
</asp:ListItem>
</asp:DropDownList>
Switch to Default.aspx.cs and add the following event handler:
void Page_PreInit(object sender, EventArgs e)
{
if (Request["ChangeTheme"] != null)
Page.Theme = Request["ChangeTheme"];
}
When we run this program we can choose a theme from the dropdown list and
when the page reloads it will have the corresponding theme applied.
If we store the theme in a users profile we can use the profile value to set
the theme for every page in our application, but it is left for you to look
into how to do this.
Note: Theme switching is much more complicated in a site based
on a MasterPage as there is no theme attribute in the <%@ master> directive.
This means each page must have it's theme set individually with code to load the
appropriate theme. There are several articles online discussing this issue -
plug
'asp.net change theme masterpage' into Google.