Write code to call the web service
We are now ready to add the code into the page to make the web
service do its work.
The basic strategy is to:
- create a new instance of your web service.
- Get the parameters from your user interface elements.
- Call the web service function using the parameters.
- Copy the result into the place holder on your web page.
We will look at the three examples from Session 2 in more detail to
see this in action.
Example 1: Global Weather
With your website still open in VWD or VS identify your user
interface element. I will assume we are going to use Liverpool as the
CityName and just display the result in an <asp:Xml> element with an id
of xmlWeather. The user interface looks like this:
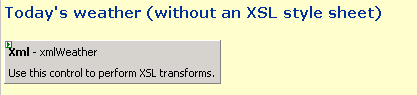
I assume you have added a web reference to your project with the name MyWeather.
You need to add a using MyWeather;
statement at the top of your .cs file.
The code to fill the Xml control with the weather value is:
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using MyWeather; // allows us to use the web reference
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// create a new object of class GlobalWeather
GlobalWeather gw = new GlobalWeather();
// call the GetWeather function and place
// the result in a string.
String localWeather = gw.GetWeather("Liverpool", "");
// Copy the returned string into the Xml control
xmlWeather.DocumentContent = localWeather;
}
}
Notice the reference to MyWeather in the using section. This allows
you to access the GlobalWeather class and create a new object from it.
The demonstration for the
GlobalWeather web service also has two enhancements. Because the
value returned is XML we can create an XSL file to transform the result
for display within our page. One example shows an XSL file which returns
several of the weather values with accompanying text. The code for this
is:
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
Location:
<xsl:value-of select="CurrentWeather/Location" /><br />
Temperature:
<xsl:value-of select="CurrentWeather/Temperature" /><br />
Wind: <xsl:value-of select="CurrentWeather/Wind" /><br />
SkyConditions:
<xsl:value-of select="CurrentWeather/SkyConditions" /><br />
Visibility:
<xsl:value-of select="CurrentWeather/Visibility" />
</xsl:template>
</xsl:stylesheet>
The last example
show the use of an XSL file to retrieve one value for placement in the
middle of the text of the page. The code for this is:
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:value-of select="CurrentWeather/Temperature" />
</xsl:template>
</xsl:stylesheet>
Example 2: Currency Converter
I assume you have added a web reference to your project and called it MyCurrency
and added a using MyCurrency;
statement at the top of your .cs file.
Using the CurrencyConvertor web service you can retrieve the conversion rate for
a whole range of currencies. The currencies are represented in an enumeration type
called Currency.
If you want to simple retrieve the conversion rate for a fixed pair of currencies
you can do this:
CurrencyConvertor curr = new CurrencyConvertor();
double rate =curr.ConversionRate
(Currency.GBP, Currency.EUR);
Label1.Text = rate.ToString();
However, if you want to allow your user to select a range of currencies the code
is a little more complicated. You can add a DropDownList and fill it manually with
the range of currencies that you want to use and then check the value that is selected.
If you set the properties of the DropDownList so that it automatically posts back
the selection when the user selects a new value you can add an event for SelectedIndexchanged
and use code to determine which currency value has been selected, like this:
CurrencyConvertor curr = new CurrencyConvertor();
Currency currType;
if (DropDownList1.SelectedValue == "EUR")
currType = Currency.EUR;
else if (DropDownList1.SelectedValue == "USD")
currType = Currency.USD;
else
currType = Currency.AUD;
double rate = curr.ConversionRate
(Currency.GBP, currType);
Label1.Text = rate.ToString();
However, this is tedious if you want the user to be able to select from the full list of currencies. We can automatically fill the DropDownList with all the currency values when the page first loads. We can then use code to convert the string value selected in the DropDownList to the correct Currency value. However, the code is quite complex, even though it is only one line of code.
The code for filling the DropDownList is:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
DropDownList1.Items.Clear();
foreach (String currency in Enum.GetNames(typeof(Currency)))
DropDownList1.Items.Add(currency);
}
}
The code for retrieving the correct currency from the value in the DropDownList is:
protected void DropDownList1_SelectedIndexChanged
(object sender, EventArgs e)
{
CurrencyConvertor curr = new CurrencyConvertor();
Currency currType =
(Currency)Enum.Parse(typeof(Currency),
DropDownList1.SelectedValue, true);
double rate = curr.ConversionRate(Currency.GBP, currType);
Label1.Text = rate.ToString();
}
The
demonstration of
CurrencyConvertor shows all three of the above techniques in operation.
Example 3: Language Translation
The TranslateService web service has a very simple interface. If we want to use
a fixed translation we simply call the service with the corresponding code. The
languages are specified using an enumeration type called Language. For example,
we can specify translation from English to German using the value Language.EnglishTOGerman.
I assume that you have already added the web reference and called it MyLanguage
and added a using MyLanguage;
statement at the top of the .cs file.
The code for a fixed translation from English to German looks like this:
TranslateService trans = new TranslateService();
Label1.Text = trans.Translate
(Language.EnglishTOGerman, TextBox1.Text);
If we want to give the user a choice we can use a similar method to the Currency
example to allow the user to select from a range of values in a DropDownList. For
example:
TranslateService trans = new TranslateService();
if (DropDownList1.SelectedValue == "English to French")
Label1.Text = trans.Translate
(Language.EnglishTOFrench, TextBox1.Text);
if (DropDownList1.SelectedValue == "English to Spanish")
Label1.Text = trans.Translate
(Language.EnglishTOSpanish, TextBox1.Text);
if (DropDownList1.SelectedValue == "French to English")
Label1.Text = trans.Translate
(Language.FrenchTOEnglish, TextBox1.Text);
if (DropDownList1.SelectedValue == "Spanish to English")
Label1.Text = trans.Translate
(Language.SpanishTOEnglish, TextBox1.Text);
The demonstration of
TranslateService shows this in action.