Displaying media from a database
There is often a requirement to deliver media content based on information
held on a database. The Embedded Media tutorial discuss the techniques used for
embedding media content as static, standards compliant xhtml.
These notes will discuss how to take the XHTML code and implement it
within a data aware server control. For this example we will use Windows
Media Video files, though the principle can be applied for any media type by
using the appropriate XHTML template code.
Download a working copy of this example.
Scenario
Assume we have an SQL database 'media.mdf' containing a table 'tblMovies'
with the following field layout:
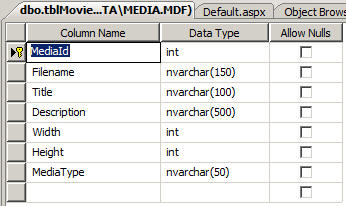
In this case 'MediaId' is an auto generated key field and is used to
identify the media files which are held in a folder called 'media'. The
original filename of each media item is held in the 'Filename' field. The 'MediaType'
field is not used in this example and is set to 'wmv' for all records. The
layout of the solution, with a simple 'default.aspx' home page and some
sample data files is shown below:
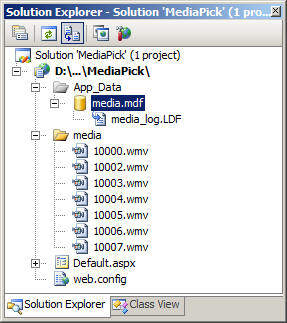
Basic Plan
For this example we will have a simple drop down list linked to the data
table displaying the titles of the videos, and a FormView control displaying
the detail of the selected video. To get started put a heading on the home
page followed by a drop down list (ddlMovies) with a suitable label and a
FormView control (frmMovie). For example:
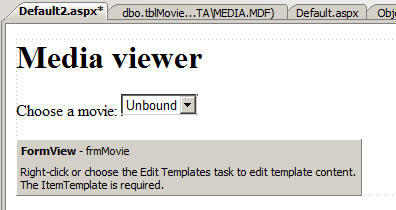
Linking to the database
For the DropDownList connect a data source to tblMovies, selecting just
the MediaId and Title fields and choose the Title field for the data field
to display in the DropDownList and the MediaId field for the data field for
the value of the DropDownList. You also need to set the AutoPostBack
property of the DropDownList to true.
For the FormView connect to the database using a new SQLDataSource and
select all the fields. Use the 'WHERE...' button to link the MediaId filed
to the ddlMovies control. You will end up with a default template for the
FormView displaying each of the fields as follows:
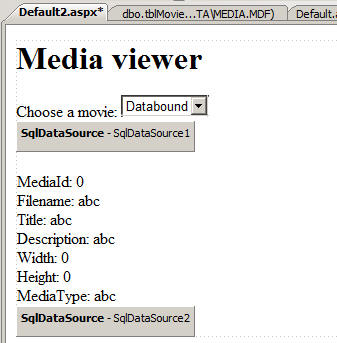
When you run the application you get a typical Master/Detail form as
follows:
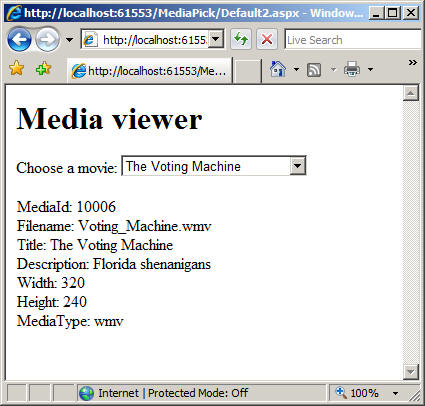
Modifying the FormView to display media
The basic XHTML for displaying a WMV file is:
<object type="video/x-ms-wmv" data="media/filename.WMV"
width="320" height="260">
<param name="src" value="media/filename.WMV" />
<param name="autoStart" value="False" />
<param name="showControls" value="True" />
<p>Cannot play WMV files!</p>
</object>
The above code shows elements highlighted where they would vary from one media record
to another.
In this simple example we only neeed to have an ItemTemplate for the
FormView, so we can delete the automatically generated EditItemTemplate and
InsertItemTemplate. We end up with:
<asp:FormView ID="frmMovie" runat="server"
DataKeyNames="MediaId"
DataSourceID="SqlDataSource2">
<ItemTemplate>
MediaId:
<asp:Label ID="MediaIdLabel" runat="server"
Text='<%# Eval("MediaId") %>' /><br />
Filename:
<asp:Label ID="FilenameLabel" runat="server"
Text='<%# Bind("Filename") %>' /><br />
Title:
<asp:Label ID="TitleLabel" runat="server"
Text='<%# Bind("Title") %>' /><br />
Description:
<asp:Label ID="DescriptionLabel" runat="server"
Text='<%# Bind("Description") %>' /><br />
Width:
<asp:Label ID="WidthLabel" runat="server"
Text='<%# Bind("Width") %>' /><br />
Height:
<asp:Label ID="HeightLabel" runat="server"
Text='<%# Bind("Height") %>' /><br />
MediaType:
<asp:Label ID="MediaTypeLabel" runat="server"
Text='<%# Bind("MediaType") %>' /><br />
</ItemTemplate>
</asp:FormView>
Note the ItemTemplate is just plain XHTML and Text interspersed with <%#
Eval("fieldname") %> elements for dropping values from the database - note
some use Bind("fieldname") instead of Eval(). We need to replace the
template contents with our XHTML and drop the field references into the
highlighted positions. We use Eval as it is simple values.
We will include the Title (as a sub-heading) and the filename and description in a
paragraph too, just to make the layout a little more realistic. We get:
<h2><%# Eval("Title") %></h2>
<p>Filename: <%# Eval("Filename") %>
- <%# Eval("Description") %></p>
<object type="video/x-ms-wmv"
data='media/<%# Eval("MediaId") %>.wmv'
width='<%# Eval("Width") %>' height='<%# Eval("Height") %>'>
<param name="src" value='media/<%# Eval("MediaId") %>.wmv' />
<param name="autoStart" value="False" />
<param name="showControls" value="True" />
<p>Unable to display video!</p>
</object>
Note the use of single quotes for attributes in the <object> and <param>
elements as the field syntax needs to use double quotes.
When we run the application we get:
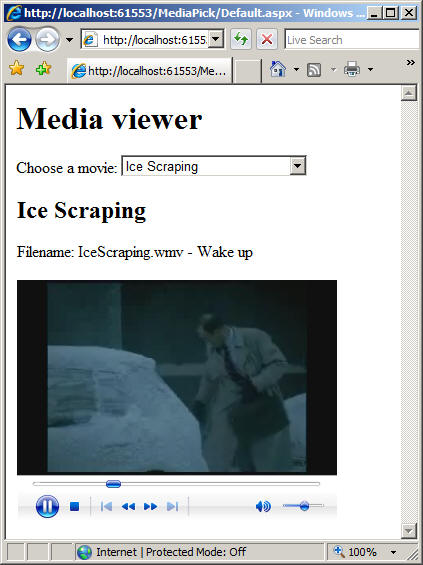
Enhancements
Now we have a template for displaying our media (and associated data) we
can use in any data bound control which supports templated design.
If we want to upload media we can use the
PicPick example as the basis for
creating an upload page.